Lifecycle
The application lifecycle has four states:
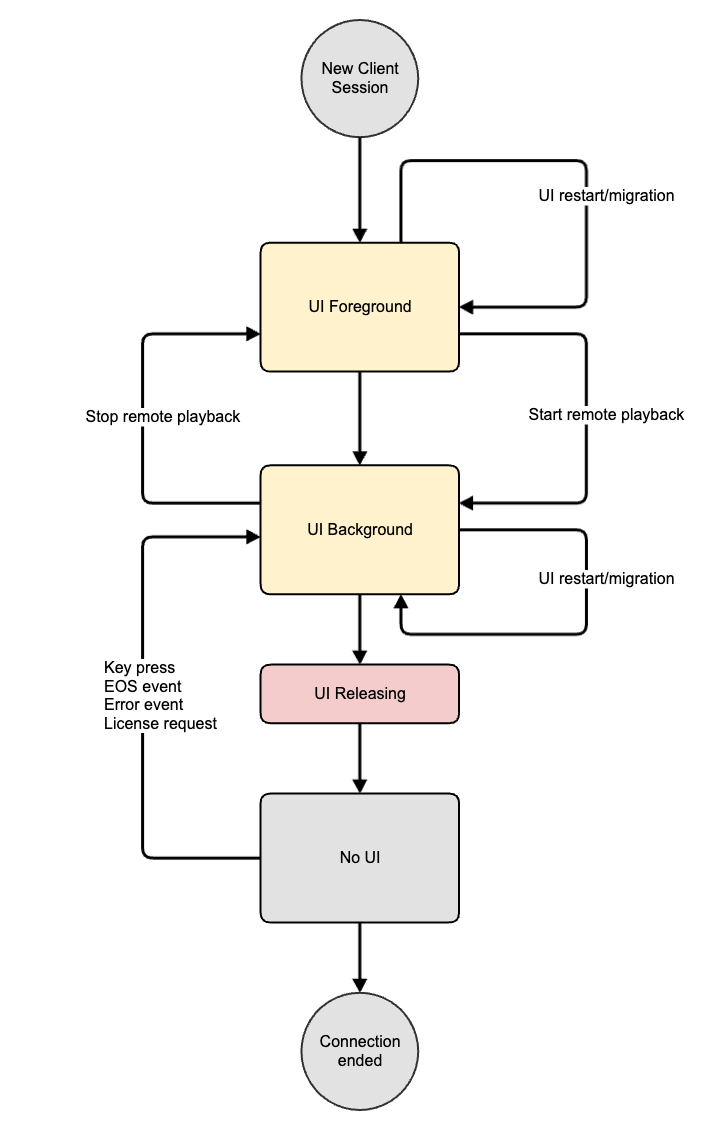
- Foreground: when the user interface is displayed
- Background: when the remote player is playing full screen video
- Transition to Foreground: in process of switching to the user interface
- Transition to Background: in process of switching to the remote player
State Management
In background mode, after a period of time the application is unloaded to save resources. It will be reloaded on the next key press, end-of-stream event, error event or request of a DRM license (either content or renewal).
In order for the user to resume where they left off, your app should preserve its state as follows:
- Before switching to the background, save the current state to local storage or session storage.
- After switching to the foreground, restore the state from local or session storage if the app has been restarted.
State Storage
See the guide on Preserving State to learn more about persistency of storage across UI release and reboots.
The sections below explain the Client Library APIs for App Lifecycle management.
Modules
Classes
- Lifecycle
Lifecycle is a singleton class that manages the application lifecycle states.
Typedefs
- ConnectReason :
Object
The reason the ui app has been loaded
- UiState :
Object
The ui lifecycle state
lifecycle β Lifecycle
Lifecycle
Returns: Lifecycle
- pointer to the Lifecycle singleton
Example
import { lifecycle } from "senza-sdk";
Lifecycle
Lifecycle is a singleton class that manages the application lifecycle states.
Kind: global class
Emits: event:onstatechange
, event:userinactivity
, event:userdisconnected
- Lifecycle
- .state β
UiState
- .connectReason β
ConnectReason
- .triggerEvent β
Object
.autoBackground :boolean
.autoBackgroundDelay :integer
.autoBackgroundOnUIDelay :integer
- .configure(config)
- .getConfiguration() β
Object
- ._moveToUiStandby()
.getState() βUiState
- .moveToForeground() β
Promise
- .moveToBackground() β
Promise
- .switchTenant(tenantId) β
Promise
- .exitApplication() β
Promise
- .addEventListener(type, listener, options)
- .removeEventListener(type, listener, options)
- "onstatechange"
- "userinactivity"
- "userdisconnected"
- .state β
lifecycle.state β UiState
UiState
Getter for returning the ui lifecycle state
Kind: instance property of Lifecycle
Returns: UiState
- the current application lifecycle state
lifecycle.connectReason β ConnectReason
ConnectReason
Getter for returning the application connection reason
Kind: instance property of Lifecycle
Returns: ConnectReason
- the application connection reason
lifecycle.triggerEvent β Object
Object
Getter for returning the event that triggered the reloading of the ui after ui has been released
Kind: instance property of Lifecycle
Returns: Object
- trigger event
Properties
Name | Type | Description |
---|---|---|
type | string | the type of the trigger event (e.g. keyPressEvent, videoPlaybackEvent) |
data | object | data of the event, dependent on its type (e.g. keyPressEvent has data of keyValue) |
lifecycle.autoBackground : boolean
boolean
Deprecated
Kind: instance property of Lifecycle
See: configure
lifecycle.autoBackgroundDelay : integer
integer
Deprecated
Kind: instance property of Lifecycle
Default: 30
See: configure
lifecycle.autoBackgroundOnUIDelay : integer
integer
Deprecated
Kind: instance property of Lifecycle
Default: -1
See: configure
lifecycle.configure(config)
Configure lifecycle settings
Kind: instance method of Lifecycle
Param | Type | Default | Description |
---|---|---|---|
config | Object | Configuration object | |
[config.autoBackground] | Object | Auto background settings | |
[config.autoBackground.enabled] | boolean | Enable/disable auto background | |
[config.autoBackground.timeout] | Object | Timeout settings | |
[config.autoBackground.timeout.playing] | number | false | 30 | Timeout in seconds when video is playing, false to disable |
[config.autoBackground.timeout.idle] | number | false | false | Timeout in seconds when in UI mode, false to disable |
lifecycle.getConfiguration() β Object
Object
Get the current configuration settings
Kind: instance method of Lifecycle
Returns: Object
- The current configuration object
Example
const config = lifecycle.getConfiguration();
console.log(config.autoBackground.enabled); // true/false
console.log(config.autoBackground.timeout.playing); // 30
console.log(config.autoBackground.timeout.idle); // false
lifecycle._moveToUiStandby()
This method moves the application into standby mode, i.e. last ui frame is displayed and ui resources are released.
It should be called whenever the application wishes to go into standby mode and release resources.
Kind: instance method of Lifecycle
Example
lifecycle._moveToUiStandby();
lifecycle.getState() β UiState
UiState
Deprecated
Kind: instance method of Lifecycle
Returns: UiState
- the current application lifecycle state
Example
try {
const state = await lifecycle.getState();
console.log("current state is", state);
} catch (e) {
console.error("getState failed", e);
}
lifecycle.moveToForeground() β Promise
Promise
Once playback starts on the remote player,
the application is moved from foreground to inTransitionToBackground and eventually to background.
The application will need to call moveToForeground when it receives an event that needs the UI to be displayed again,
for example a key press, a playback end-of-file or a playback error.
Kind: instance method of Lifecycle
Returns: Promise
- Promise which is resolved when the moveToForeground command has been successfully processed.
Failure to process the moveToForeground command will result in the promise being rejected.
lifecycle.moveToBackground() β Promise
Promise
This method moves the application to the background.
It should be called after remotePlayer.play().
As a consequence, remote player playback will be displayed in full screen.
Kind: instance method of Lifecycle
Returns: Promise
- Promise which is resolved when the moveToBackground command has been successfully processed.
Failure to process the moveToBackground command will result in the promise being rejected.
Example
remotePlayer.load("https://example.com/video.mp4", 0);
remotePlayer.play();
lifecycle.moveToBackground();
lifecycle.switchTenant(tenantId) β Promise
Promise
Use this api to switch to another tenant (other than the home tenant) which will launch the application associated with the tenantId. The tenantId must be configured in the
Senza platform. Switching to the home tenant should use the exitApplication().
Kind: instance method of Lifecycle
Returns: Promise
- Promise which is resolved when the switchTenant command has been successfully processed.
Failure to process the switchTenant command will result in the promise being rejected.
Param | Type | Description |
---|---|---|
tenantId | string | The tenantId to switch |
lifecycle.exitApplication() β Promise
Promise
Use this api to exit the application which will redirect the browser to the home tenant application.
Kind: instance method of Lifecycle
Returns: Promise
- Promise which is resolved when the exitApplication command has been successfully processed.
Failure to process the exitApplication command will result in the promise being rejected.
lifecycle.addEventListener(type, listener, options)
Add event listener for lifecycle events
Kind: instance method of Lifecycle
Param | Type | Description |
---|---|---|
type | string | The event type to listen for |
listener | function | The callback function. Listeners for 'userdisconnected' events should return a promise to ensure the event is processed before the application exits. |
options | Object | Event listener options |
lifecycle.removeEventListener(type, listener, options)
Remove event listener
Kind: instance method of Lifecycle
Param | Type | Description |
---|---|---|
type | string | The event type |
listener | function | The callback function to remove |
options | Object | Event listener options |
"onstatechange"
Fired after transition from one state to another.
The flow is: foreground --> inTransitionToBackground --> background --> inTransitionToForeground --> foreground
Kind: event emitted by Lifecycle
Properties
Name | Type | Description |
---|---|---|
state | UiState | Indicates the new state. |
Example
lifecycle.addEventListener("onstatechange", (e) => {
console.log("new state is", e.state);
});
"userinactivity"
Fired after the ui has been inactive (i.e. no key presses) for a configurable number of seconds.
Kind: event emitted by Lifecycle
Alpha: API has not yet been released
Properties
Name | Type | Description |
---|---|---|
timeout | number | the number of seconds after which the application will be unloaded. |
Example
lifecycle.addEventListener("userinactivity", (e) => {
console.log(`Application will be unloaded in ${e.timeout} seconds`);
});
"userdisconnected"
Fired when the user session ends .
This event is useful for cleaning up application state or saving data before the application closes. Event callback should return promise to ensure that the event is handled before the application is terminated
Kind: event emitted by Lifecycle
Example
lifecycle.addEventListener("userdisconnected", () => {
console.log("User session ended, cleaning up application state");
// Perform any necessary cleanup here
});
ConnectReason : Object
Object
The reason the ui app has been loaded
Kind: global typedef
Properties
Name | Type | Description |
---|---|---|
UNKNOWN | string | |
INITIAL_CONNECTION | string | Indicates that ui app has been loaded for the first time |
APPLICATION_RELOAD | string | Indicates that ui app has been reloaded (e.g. after HOME keypress) |
UI_RELEASE | string | Indicates that ui app has been reloaded after ui release |
UI_TERMINATION | string | Indicates that ui app has been reloaded due to ui termination |
WEBRTC_ERROR | string | Indicates that ui app has been reloaded due to webrtc error |
UI_WATCHDOG | string | Indicates that ui app has been reloaded due to ui watchdog not receiving ui frames |
UiState : Object
Object
The ui lifecycle state
Kind: global typedef
Properties
Name | Type | Description |
---|---|---|
UNKNOWN | string | state is unknown at this time |
FOREGROUND | string | ui is displayed |
IN_TRANSITION_TO_FOREGROUND | string | ui is about to be displayed |
BACKGROUND | string | remote player is playing (full screen playback is displayed) |
IN_TRANSITION_TO_BACKGROUND | string | remote player is about to be playing |
Updated 3 months ago