Geolocation
Show content that depends upon the device's location
https://youtu.be/rc75GhrWpe8https://youtu.be/rc75GhrWpe8Web servers typically have the ability to use the client's IP address to determine the user's location. They may do this to provide a customized experience to users in different countries, or to limit who can connect to a service.
With Senza, the web app runs in a browser in the cloud. When the app makes a request to a server, it will appear to have an IP address that corresponds to the location of the cloud provider's data center. For example, Amazon's us-east-1 data center is in Ashburn, Virgina. By default, if you were to attempt to geolocate users running an app deployed on a tenant in this data center, they would all appear to be in the United States.
The Device Manager object in the client library has a deviceInfo
property that contains two IP address values, as well as the country code:
remoteBrowserIp
: the address of the browser is running in the cloud.clientIp
: the address of the physical device, or the computer running the simulator.countryCode
: the two-letter code of the country where the device is located.
The remote browser IP is the same one that a web server would normally see, while the client IP is the one that you'll probably find more useful. If all you need is the country, this is already provided in the device info.
- Source code: https://github.com/synamedia-senza/flags/
- Demo: http://andrewzc.net/flags/
Video tutorial
Warning: contains silly accents.
App
Our app will geolocate the device and display the flag of the country where it is located.
Start with some HTML that has a single fullscreen image tag:
<!DOCTYPE html>
<html>
<head>
<title>Flags</title>
</head>
<body style="margin: 0px; background-color: black; overflow: hidden;">
<img id="image" src="" width="1920" height="1080">
</body>
<script src="https://senza-sdk.streaming.synamedia.com/latest/bundle.js"></script>
<script type="text/javascript" src="config.js"></script>
<script type="text/javascript" src="flags.js"></script>
</html>
We won't need a separate CSS file for this app since it is so simple.
Geolocation
The entire program is as follows. The important bit is really just a single line of code!
- Get the country code from the Device Manager's
deviceInfo
object. - Alternatively, if you want more information than just the country, you can look up the IP address yourself:
- Get the client IP address from the Device Manager's
deviceInfo
object. - Call the ipdata.io API to look up the IP address and get the JSON response.
- Get the two-letter country code from the response.
- Get the client IP address from the Device Manager's
- Set the image source to a flag from flagpedia.net.
window.addEventListener("load", async () => {
await senza.init();
let countryCode = senza.deviceManager.deviceInfo.countryCode;
// Alternatively, if you want to look up the IP address yourself:
// let ipAddress = senza.deviceManager.deviceInfo.clientIp;
// let ipdata = await (await fetch(`https://api.ipdata.co/${ipAddress}?api-key=${API_KEY}`)).json();
// let countryCode = ipdata.country_code;
image.src = `https://flagpedia.net/data/flags/w1160/${countryCode.toLowerCase()}.webp`;
});
If you prefer to use ipdata.io to geolocate IP addresses, then you can create an account, request an API key, and enter it in the config.js
file:
// Get an API key from ipdata.co
const API_KEY = "your-api-key";
That's it!
Testing
When you run the program on a device, it will show you the flag for the country where the device is located. When you run the program in the Simulator, it will do the same for where your computer is located.
Would you like to see how your app would look in another country? In the Simulator, you can override the country reported by the device manager. Choose More > Settings, and then select a country from the menu. When you connect again, it will report the code for the country that you selected.
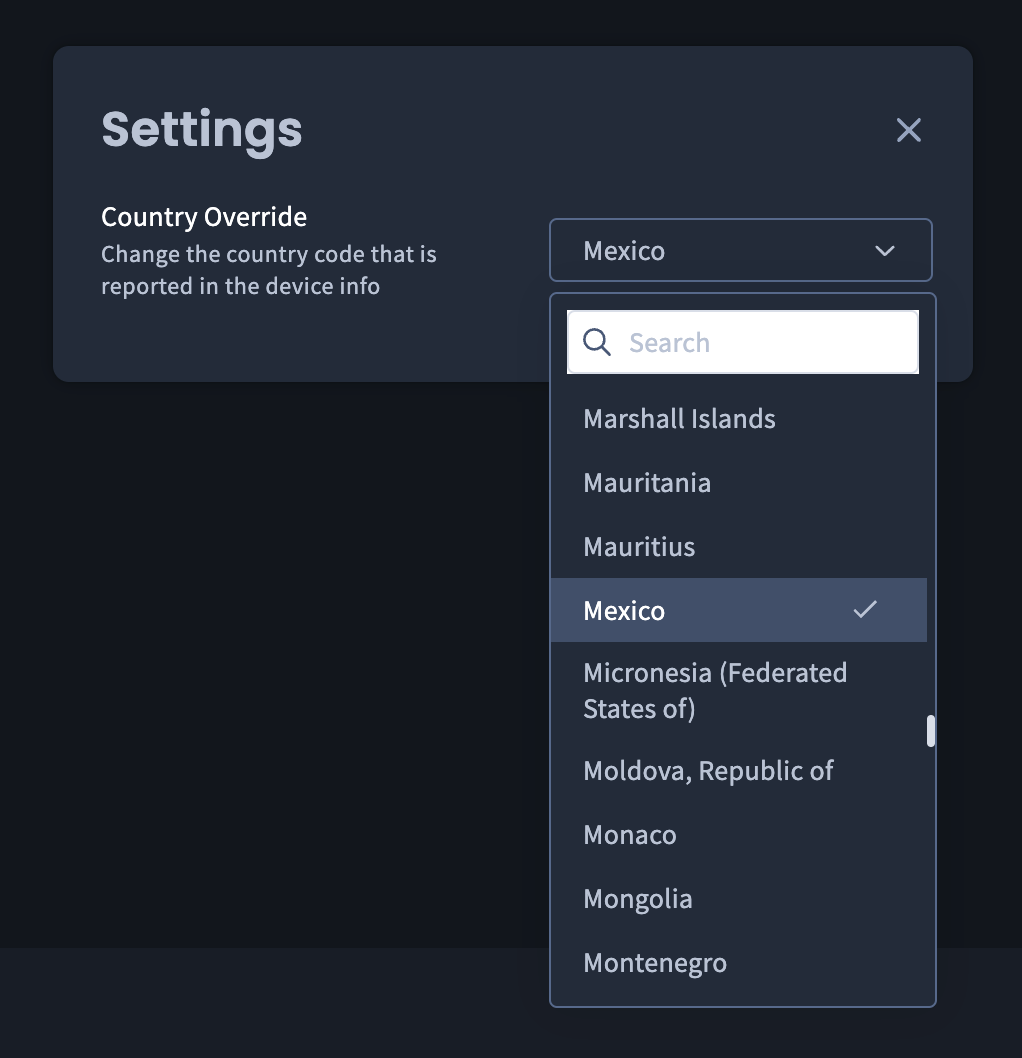
Alternatively, you can create a tunnel from your computer to a VPN provider in another country. Then if you run the app in the Simulator, both the ipAddress
and countryCode
values will reflect the location where the VPN server is located.
This wouldn't work for a physical device, unless you have a hardware VPN that is running at the network level.
Conclusion
Now that you've seen how to determine the country of the user's device, you can build this very short amount of code into your app to provide them with a customized experience, or restrict which users can access your content.
Updated about 2 months ago